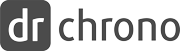
Drchrono.com v4 (Hunt Valley)
Added: 15/04/2021
Updated at: 12/07/2021
This document is intended as a detailed reference for the precise behavior of the drchrono API. If this is your first time using the API, start with our tutorial. If you are upgrading from a previous version, take a look at the changelog section. # Authorization ## Initial authorization There are three main steps in the OAuth 2.0 authentication workflow: 1. Redirect the provider to the authorization page. 2. The provider authorizes your application and is redirected back to your web application. 3. Your application exchanges the `authorization_code` that came with the redirect for an `access_token` and `refresh_token`. ### Step 1: Redirect to drchrono The first step is redirecting your user to drchrono, typically with a button labeled "Connect to drchrono" or "Login with drchrono". This is just a link that takes your user to the following URL: https://drchrono.com/o/authorize/?redirect_uri=REDIRECT_URI_ENCODED&response_type=code&client_id=CLIENT_ID_ENCODED&scope=SCOPES_ENCODED - `REDIRECT_URI_ENCODED` is the URL-encoded version of the redirect URI (as registered for your application and used in later steps). - `CLIENT_ID_ENCODED` is the URL-encoded version of your application's client ID. - `SCOPES_ENCODED` is a URL-encoded version of a space-separated list of scopes, which can be found in each endpoint or omitted to default to all scopes. The `scope` parameter consists of an optional, space-separated list of scopes your application is requesting. If omitted, all scopes will be requested. Scopes are of the form `BASE_SCOPE:[read|write]` where `BASE_SCOPE` is any of `user`, `calendar`, `patients`, `patients:summary`, `billing`, `clinical` and `labs`. You should request only the scopes you need. For instance, an application which sends "Happy Birthday!" emails to a doctor's patients on their birthdays would use the scope parameter `"patients:summary:read"`, while one that allows patients to schedule appointments online would need at least `"patients:summary:read patients:summary:write calendar:read calendar:write clinical:read clinical:write"`. ### Step 2: Provider authorization After logging in (if necessary), the provider will be presented with a screen with your application's name and the list of permissions you requested (via the `scope` parameter). When they click the "Authorize" button, they will be redirected to your redirect URI with a `code` query parameter appended, which contains an authorization code to be used in step 3. If they click the "Cancel" button, they will be redirected to your redirect URI with `error=access_denied` instead. Note: This authorization code expires extremely quickly, so you must perform step 3 immediately, ideally before rendering the resulting page for the end user. ### Step 3: Token exchange The `code` obtained from step 2 is usable exactly once to obtain an access token and refresh token. Here is an example token exchange in Python: import datetime, pytz, requests if 'error' in get_params: raise ValueError('Error authorizing application: %s' % get_params[error]) response = requests.post('https://drchrono.com/o/token/', data={ 'code': get_params['code'], 'grant_type': 'authorization_code', 'redirect_uri': 'http://mytestapp.com/redirect_uri', 'client_id': 'abcdefg12345', 'client_secret': 'abcdefg12345', }) response.raise_for_status() data = response.json() # Save these in your database associated with the user access_token = data['access_token'] refresh_token = data['refresh_token'] expires_timestamp = datetime.datetime.now(pytz.utc) + datetime.timedelta(seconds=data['expires_in']) You now have all you need to make API requests authenticated as that provider. When using this access token, you'll only be able to access the data that the user has access to and that you have been granted permissions for. ## Refreshing an access token Access tokens only last 48 hours (given in seconds in the `'expires_in'` key in the token exchange step above), so they occasionally need to be refreshed. It would be inconvenient to ask the user to re-authorize every time, so instead you can use the refresh token like the original authorization to obtain a new access token. Replace the `code` parameter with `refresh_token`, change the value `grant_type` from `authorization_code` to `refresh_token`, and omit the `redirect_uri` parameter. Example in Python: ... response = requests.post('https://drchrono.com/o/token/', data={ 'refresh_token': get_refresh_token(), 'grant_type': 'refresh_token', 'client_id': 'abcdefg12345', 'client_secret': 'abcdefg12345', }) ... # Webhooks In order to use drchrono API webhooks, you first need to have an API application on file (even if it is in Test Model). Each API webhook is associated with one API application, go to here to set up both API applications and webhooks! Once you registered an API application, you will see webhook section in each saved API applications. Create a webhook and register some events there and save all the changes, then you are good to go! ## Webhooks setup All fields under webhooks section are required. **Callback URL** Callback URl is used to receive all hooks when subscribed events are triggered. This should be an URL under your control. **Secret token** Secret token is used to verify webhooks, this is very important, please set something with high entropy. Also we will talk more about this later. **Events** Events is used to register events you want to receiver notification when they happen. Currently we support following events. Event name | Event description ---------- | ----------------- `APPOINTMENT_CREATE` | We will deliver a hook any time an appointment is created `APPOINTMENT_MODIFY` | We will deliver a hook any time an appointment is modified `PATIENT_CREATE` | We will deliver a hook any time a patient is created `PATIENT_MODIFY` | We will deliver a hook any time a patient is modified `PATIENT_PROBLEM_CREATE` | We will deliver a hook any time a patient problem is created `PATIENT_PROBLEM_MODIFY` | We will deliver a hook any time a patient problem is modified `PATIENT_ALLERGY_CREATE` | We will deliver a hook any time a patient allergy is created `PATIENT_ALLERGY_MODIFY` | We will deliver a hook any time a patient allergy is modified `PATIENT_MEDICATION_CREATE` | We will deliver a hook any time a patient medication is created `PATIENT_MEDICATION_MODIFY` | We will deliver a hook any time a patient medication is modified `CLINICAL_NOTE_LOCK` | We will deliver a hook any time a clinical note is locked `CLINICAL_NOTE_UNLOCK` | We will deliver a hook any time a clinical note is unlocked `TASK_CREATE` | We will deliver a hook any time a task is created `TASK_MODIFY` | We will deliver a hook any time a task is modified and any time creation, modification and deletion of task notes, associated task item `TASK_DELETE` | We will deliver a hook any time a task is deleted ## Webhooks verification In order to make sure the callback URL in webhook is under your control, we added a verification step before we send any hooks out to you. Verification can be done by clicking "Verify webhook" button in webhooks setup page. After you click the button, we will send a `GET` request to the callback URL, along with a parameter called `msg`. Please use your webhook's secret token as hash key and SHA-256 as digest constructor, hash the `msg` value with HMAC algorithm. And we expect a `200` JSON response, in JSON response body, there should be a key called `secret_token` existing, and its value should be equal to the hashed `msg`. Otherwise, verification will fail. Here is an example webhook verification in Python: import hashlib, hmac def webhook_verify(request): secret_token = hmac.new(WEBHOOK_SECRET_TOKEN, request.GET['msg'], hashlib.sha256).hexdigest() return json_response({ 'secret_token': secret_token })
secret_token
is needed in
request header.
Try to set the secret_token
to something with high entropy, a good example could be taking the output of
ruby -rsecurerandom -e 'puts SecureRandom.hex(20)'
.
After this, you might want to verify all request headers you received on your server with this token.
# iframe integration
Some API apps provide additional functionality for interacting with patient data
not offered by drchrono, and can benefit by being incorporated into drchrono's
patient information page via iframe. We have created a simple API to make this
possible.
To make an existing API application accessible via an iframe on the patient
page, you need to update either "Patient iframe" or "Clinical note iframe" section in API management page,
to make the iframe to appear on (either the patient page or the clinical note page),
with the URL that the iframe will use for each page, and the height it should
have. The application will be reviewed before it is approved to ensure that it
is functional and secure.
## Register a Doctor
iframe applications will appear as choices on the left-hand menu of the patient
page for doctors registered with your application. To register a doctor with
your application, make a `POST` request to the `/api/iframe_integration`
endpoint using the access token for the corresponding doctor. This endpoint does not
expect any payload.
To disable your iframe application for a doctor, make a `DELETE` request to the
same endpoint.
## Populating the iframe
There are two places where the iframe can be displayed, either within the
patient detail page or the clinical note page, shown below respectively:

